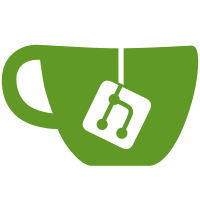
Document.execCommand() has been deprecated and altthough some browsers might still support it, it may have already been removed from the relevant web standards, may be in the process of being dropped, or may only be kept for compatibility purposes, my alternative uses instead the Clipboard API to copy the generated password to the clipboard
73 lines
2.1 KiB
JavaScript
73 lines
2.1 KiB
JavaScript
const resultEl = document.getElementById('result')
|
|
const lengthEl = document.getElementById('length')
|
|
const uppercaseEl = document.getElementById('uppercase')
|
|
const lowercaseEl = document.getElementById('lowercase')
|
|
const numbersEl = document.getElementById('numbers')
|
|
const symbolsEl = document.getElementById('symbols')
|
|
const generateEl = document.getElementById('generate')
|
|
const clipboardEl = document.getElementById('clipboard')
|
|
|
|
const randomFunc = {
|
|
lower: getRandomLower,
|
|
upper: getRandomUpper,
|
|
number: getRandomNumber,
|
|
symbol: getRandomSymbol
|
|
}
|
|
|
|
clipboardEl.addEventListener('click', () => {
|
|
const password = resultEl.innerText;
|
|
if (!password) {
|
|
return;
|
|
}
|
|
navigator.clipboard.writeText(password);
|
|
alert('Password copied to clipboard!')
|
|
})
|
|
|
|
generateEl.addEventListener('click', () => {
|
|
const length = +lengthEl.value
|
|
const hasLower = lowercaseEl.checked
|
|
const hasUpper = uppercaseEl.checked
|
|
const hasNumber = numbersEl.checked
|
|
const hasSymbol = symbolsEl.checked
|
|
|
|
resultEl.innerText = generatePassword(hasLower, hasUpper, hasNumber, hasSymbol, length)
|
|
})
|
|
|
|
function generatePassword(lower, upper, number, symbol, length) {
|
|
let generatedPassword = ''
|
|
const typesCount = lower + upper + number + symbol
|
|
const typesArr = [{lower}, {upper}, {number}, {symbol}].filter(item => Object.values(item)[0])
|
|
|
|
if(typesCount === 0) {
|
|
return ''
|
|
}
|
|
|
|
for(let i = 0; i < length; i += typesCount) {
|
|
typesArr.forEach(type => {
|
|
const funcName = Object.keys(type)[0]
|
|
generatedPassword += randomFunc[funcName]()
|
|
})
|
|
}
|
|
|
|
const finalPassword = generatedPassword.slice(0, length)
|
|
|
|
return finalPassword
|
|
}
|
|
|
|
function getRandomLower() {
|
|
return String.fromCharCode(Math.floor(Math.random() * 26) + 97)
|
|
}
|
|
|
|
function getRandomUpper() {
|
|
return String.fromCharCode(Math.floor(Math.random() * 26) + 65)
|
|
}
|
|
|
|
function getRandomNumber() {
|
|
return String.fromCharCode(Math.floor(Math.random() * 10) + 48)
|
|
}
|
|
|
|
function getRandomSymbol() {
|
|
const symbols = '!@#$%^&*(){}[]=<>/,.'
|
|
return symbols[Math.floor(Math.random() * symbols.length)]
|
|
}
|